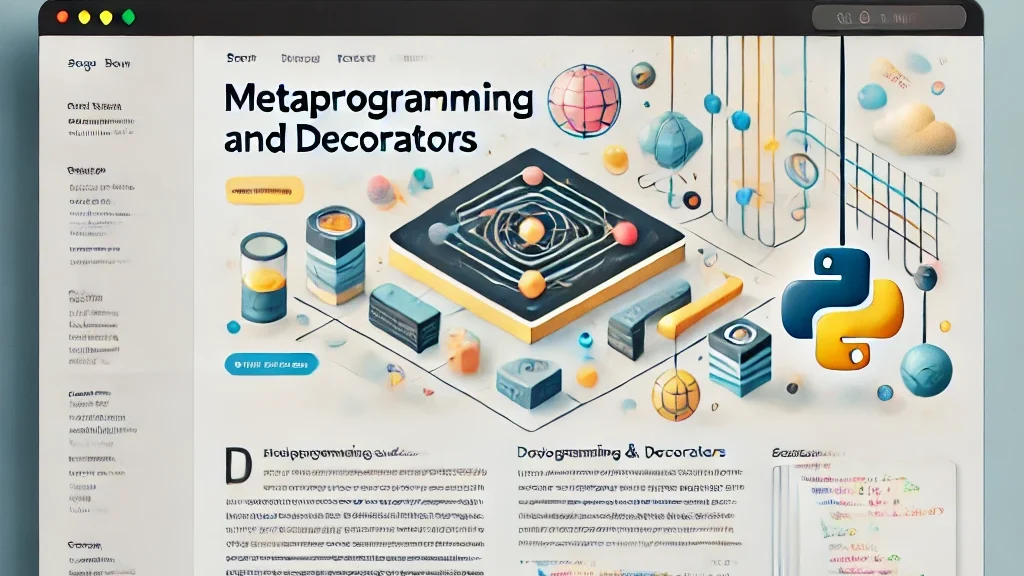
Asynchronous programming is a core part of modern web development, allowing applications to handle multiple tasks concurrently without blocking the main thread. JavaScript, being single-threaded, leverages asynchronous programming to improve performance, particularly in web browsers, where you don’t want the UI to freeze while tasks such as network requests, file reading, or long computations are happening. In this post, we will explore advanced concepts around asynchronous programming in JavaScript, including callbacks, promises, async/await, and best practices for using them in real-world applications.
The Evolution of Asynchronous JavaScript
JavaScript’s asynchronous handling evolved over time, starting with callbacks and moving to promises and eventually async/await. Let’s take a look at each.
1. Callbacks: The Original Asynchronous Solution
Callbacks were the first approach used to handle asynchronous code in JavaScript. The idea is simple: pass a function (the callback) to be executed after a task is completed.
Here’s an example using a callback:
function fetchData(callback) {
setTimeout(() => {
console.log('Data fetched');
callback('Data');
}, 1000);
}
fetchData((data) => {
console.log(`Callback received: ${data}`);
});
In the example above, the fetchData
function simulates an asynchronous task (using setTimeout
) and then calls the callback
function once the task is complete.
While this works, there are major downsides:
- Callback Hell: If there are many asynchronous operations, callbacks can become deeply nested, making code harder to read and maintain.
- Error Handling: Handling errors in callbacks can be cumbersome and lead to duplicated logic.
2. Promises: A Cleaner Approach
Promises were introduced in ES6 to handle asynchronous code more elegantly. A promise represents a value that may be available now, or in the future, or never.
Here’s the same example using promises:
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
console.log('Data fetched');
resolve('Data');
}, 1000);
});
}
fetchData()
.then((data) => {
console.log(`Promise received: ${data}`);
})
.catch((error) => {
console.error(`Error: ${error}`);
});
Benefits of Promises:
- Chaining: You can chain
.then()
calls to handle multiple asynchronous tasks in sequence. - Error Handling: Promises provide
.catch()
for centralized error handling, which simplifies error management in complex scenarios.
However, while promises were a step up from callbacks, there were still some cases where managing asynchronous code became difficult, especially when dealing with multiple asynchronous operations.
3. Async/Await: Syntactic Sugar Over Promises
Introduced in ES8, async/await
makes asynchronous code look and behave like synchronous code. The async
keyword is used to define a function that returns a promise, and await
pauses the execution of the function until the promise is resolved.
Here’s how the fetchData
function looks with async/await:
async function fetchData() {
return new Promise((resolve) => {
setTimeout(() => {
console.log('Data fetched');
resolve('Data');
}, 1000);
});
}
async function main() {
const data = await fetchData();
console.log(`Async/Await received: ${data}`);
}
main();
Benefits of async/await
:
- Readability: The code is much more readable and resembles synchronous code, which is easier to follow and debug.
- Error Handling: You can use
try/catch
blocks for error handling, which works just like synchronous code.
async function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
console.log('Data fetched');
resolve('Data');
}, 1000);
});
}
async function main() {
try {
const data = await fetchData();
console.log(`Async/Await received: ${data}`);
} catch (error) {
console.error(`Error: ${error}`);
}
}
main();
4. Combining async/await
with Multiple Asynchronous Tasks
Let’s take things a step further by running multiple asynchronous tasks concurrently. This is where Promise.all
comes in handy. Promise.all
waits for multiple promises to resolve and returns an array of their results.
Here’s an example:
function fetchData1() {
return new Promise((resolve) => {
setTimeout(() => {
resolve('Data 1');
}, 1000);
});
}
function fetchData2() {
return new Promise((resolve) => {
setTimeout(() => {
resolve('Data 2');
}, 1500);
});
}
async function main() {
try {
const [data1, data2] = await Promise.all([fetchData1(), fetchData2()]);
console.log(data1); // Data 1
console.log(data2); // Data 2
} catch (error) {
console.error(`Error: ${error}`);
}
}
main();
In this example, both fetchData1
and fetchData2
run concurrently. Promise.all
ensures that we wait for both to finish before continuing, and the results are logged in parallel.
5. Best Practices for Asynchronous JavaScript
While using async/await
is generally the preferred way to handle asynchronous code, there are a few best practices to keep in mind:
a. Use async/await
for Sequential Tasks
Whenever possible, prefer async/await
for handling sequential asynchronous operations, as it makes the code more readable and less prone to errors.
b. Use Promise.all
for Concurrent Tasks
When dealing with multiple independent asynchronous operations that can run concurrently, use Promise.all
to execute them simultaneously and wait for all to complete.
c. Handle Errors Gracefully
Always handle errors when working with asynchronous code, either through .catch()
with promises or try/catch
blocks with async/await
.
d. Avoid Blocking the Event Loop
Avoid operations that block the event loop. For example, use setTimeout
or setImmediate
for I/O-bound tasks, rather than long-running, synchronous operations.
Conclusion
Mastering asynchronous programming in JavaScript is crucial for writing efficient, scalable applications. While callbacks were the original way to handle asynchronous tasks, promises and async/await
provide a cleaner, more readable approach for handling asynchronous operations. By understanding how these tools work together, you can build more efficient and maintainable code that can handle complex tasks concurrently. Whether you’re building a web app with real-time features or handling network requests, mastering asynchronous JavaScript will unlock new performance potential and improve the overall user experience.